Now that we know how to get data from the SAP Datasphere API using Postman, I want to automate the steps using Python. In this post, I implement authentication via OAuth. I use the two existing libraries OAuth2Session and urllib.parse.
To create the OAuth request we need the secret, the client ID, the authorization URL and the token URL. I stored all this information in a JSON file so I can read and process it. Where to get this information can be found in the earlier post. So we need to read the JSON file first to get all the parameters.
secrets_file = path_to_the_secret.json f = open(secrets_file) secrets = json.load(f) print(secrets)
Next, we need to encode the client ID, as described by Jascha in this post. I use the urllib.parse.quote functionality for this..
client_id_encode = urllib.parse.quote(secrets['client_id'])
With the print command, you should see a result like this: sb-e4c75210-40c4-4c89-a960-381b156c4e93%21b106441%7Cclient%21b3650
Once we have the encoded client ID, we can create the URL for authentication. For this, we need the authorization URL and combine it with the client ID.
code_url = secrets['authorization_url'] + '?response_type=code&client_id=' + client_id_encode
When you print the URL, it should look like this:
https://xyz.authentication.eu10.hana.ondemand.com/oauth/authorize?response_type=code&client_id=sb-e4c75210-40c4-4c89-a960-381b156c4e93%21b106441%7Cclient%21b3650
Open the printed URL in a browser and copy the code you get at the end.
Now we can create the session object for the request.
session = requests.session()
And use an input function to store the code we get from the above url, see screenshot

This is all we need for the OAuth process with Python.
OAuth_AccessRequest = session.post( secrets['token_url'], auth=(secrets['client_id'], secrets['client_secret']), headers={"x-sap-sac-custom-auth": "true", "content-type": "application/x-www-form-urlencoded", "redirect_uri": 'http://localhost' }, data={'grant_type': 'authorization_code', 'code': code, 'response_type': 'token' } )
As you can see, we use the token URL, the client ID, the client secret, and the code we got from the URL earlier. The redirect is localhost, where we added the OAuth client. With the print statement, we can print the JSON response from the request.
print(OAuth_AccessRequest.json())

That is all the magic. We are now using Python to authenticate to the SAP Datasphere API. The next steps are to store the information for future use and to renew the token when it expires. In my example, I store the information in a file and use it to renew the token.
token = OAuth_AccessRequest.json() with open(path, 'w') as f: json.dump(token, f)
Updating the token is simple. First, we need to read the file where we previously stored the information. Open the file with the secret information, create an OAuth session object, and refresh the token.
f = open(token_file) token = json.load(f) f = open(path_of_secret_file) secrets = json.load(f) extra = { 'client_id': secrets['client_id'], 'client_secret': secrets['client_secret'] } datasphere = OAuth2Session(secrets['client_id'], token=token) token = datasphere.refresh_token(token_url=secrets['token_url'], **extra)
Conclusion
That's it. You can now authenticate to the Datasphere API using OAuth from Python. I know there's a manual step in the code, but that's not a showstopper for me right now. I only need to authenticate with the code after 30 days, and that is fine for some admin tools. If you want to automate some data extractions, then you can build something for that. Jascha described a way in this post. I look forward to hearing from you and your ideas about what we can do with Python and the API in the future. The next blog will be about processing information with Python, so stay tuned.
author.
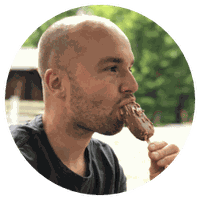
Subscribe
- In my newsletter, you get informed about new topics
- You learn how to use Analysis Office
- You get tips and tricks about SAP BI topics
- You get the first 3 chapters of my e-book Analysis Office - The Comprehensive Guide for free
You want to know SAP Analysis Office in a perfect detail?
You want to know how to build an Excel Dashboard with your Query in Analysis Office?
You want to know how functions in SAP Analysis Office works?
Then you have to take a look into Analysis Office - The Comprehensive Guide. Either as a video course or as an e-book.
Write a comment